python常用代码片段
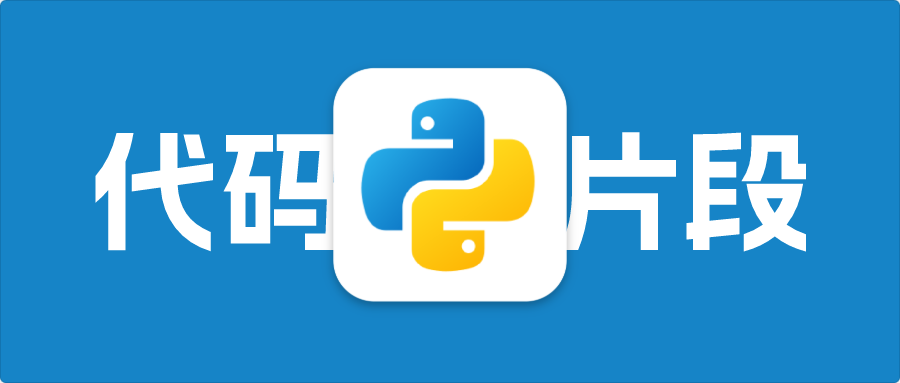
AI-摘要
Tianli GPT
AI初始化中...
介绍自己 🙈
生成本文简介 👋
推荐相关文章 📖
前往主页 🏠
前往爱发电购买
python常用代码片段
钱涛基础类
执行终端命令
1 | def subPopen(cmd: Union[str, list], shell: bool = True, encoding: str = "utf-8", errors: str = "ignore") -> str: |
网络类
获取当前wifi
1 | def getCurrentWifi() -> tuple: |
检查网络连接状态
1 | def ping(ip: str, count: int = 1, timeout: int = 100) -> bool: |
获取网络适配器IP
1 | def getNetworkAdapterIp() -> dict: |
设备类
获取设备号
1 | def getDevices() -> list: |
是否亮屏
1 | def screenOn() -> bool: |
自动接听
1 | def autoAnswer(device: str, number: str, duration: int = 10) -> None: |
根据手机号判断运营商
1 | def checkOperator(number: str) -> str: |
文件下载
https://www.taonotespace.com/download/common_python_snippets.py
评论
匿名评论隐私政策